How do you export default a function in React?
Usually, the default export is written after the function, like the example below. Copy # react function App() { return ( <div className="App"> <header className="App-header"> <img src={logo} className="App-logo" alt="logo" /> </header> </div> ); } export default App; But it can also be written like below.
How do I export all functions in React?
Use named exports to export multiple functions in React, e.g. export function A() {} and export function B() {} . The exported functions can be imported by using a named import as import {A, B} from './another-file' . You can have as many named exports as necessary in a single file.
How do I export a function within a functional component?
All you have to do is to make good use of refs. import React { useRef } from "react"; import ComponentOne from "./ComponentOne"; const ComponentTwo = () => { const componentOneRef = useRef(null); const componentOne = <ComponentOne ref={ componentOneRef } />; return ( <div> <button onClick={ () => componentOneRef.
What is a default function in React?
The defaultProps is a React component property that allows you to set default values for the props argument. If the prop property is passed, it will be changed. The defaultProps can be defined as a property on the component class itself, to set the default props for the class.
What is export default function?
Export Default is used to export only one value from a file which can be a class, function, or object. The default export can be imported with any name.
What is export default vs export?
Exports without a default tag are Named exports. Exports with the default tag are Default exports. Using one over the other can have effects on your code readability, file structure, and component organization. Named and Default exports are not React-centric ideas.
How do I export multiple functions by default?
To export multiple functions in JavaScript, use the export statement and export the functions as an object. Alternatively, you can use the export statement in front of the function definitions. This exports the function in question automatically and you do not need to use the export statement separately.
How do you export a function from one js file to another?
...
To import a function from another file in JavaScript:
- Export the function from file A , e.g. export function sum() {} .
- Import the function in file B as import {sum} from './another-file. js' .
- Use the imported function in file B .
How do you export a module from a function?
- Create a file named as app. js and export the function using module. exports . module.exports = function (a, b) { console.log(a + b); }
- Create a file named as index. js and import the file app. js to use the exported function. const sum = require( './app' ); sum(2, 5);
- Output: 7.
How do I import a function into React?
...
To import a function from another file in React:
- Export the function from file A , e.g. export function sum() {} .
- Import the function in file B as import {sum} from './another-file' .
- Use the imported function in file B .
How do I export a variable in React?
To import a variable from another file in React: Export the variable from file A , e.g. export const str = 'hello world' . Import the variable in file B as import {str} from './another-file' .
What is a React function component?
A React functional component is a simple JavaScript function that accepts props and returns a React element. After the introduction of React Hooks, writing functional components has become the standard way of writing React components in modern applications.
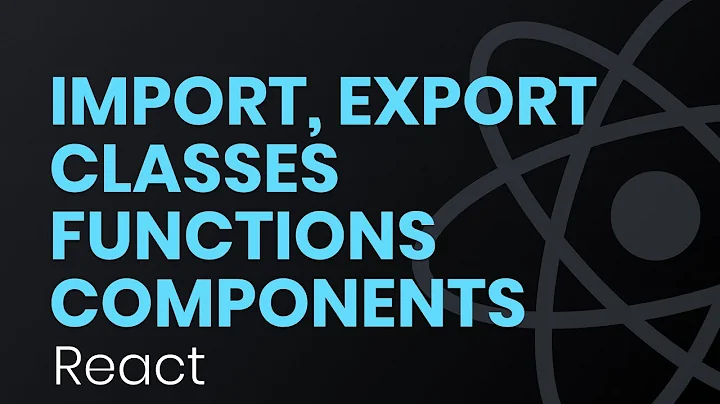
When we define the default value for a function?
6. When we define the default values for a function? Explanation: Default values for a function is defined when the function is declared inside a program.
When should I use export default?
- So, when you're exporting only one element from your module and you don't care of its name, use export default .
- If you want to export some specific element from your module and you do care of their names, use export const.
What is export function in JavaScript?
The export statement is used in Javascript modules to export functions, objects, or primitive values from one module so that they can be used in other programs (using the 'import' statement). A module encapsulates related code into a single unit of code. All functions related to a single module are contained in a file.
What are export types in Reactjs?
There are two types of exports: Named and Default.
What is export default app in React?
export default is used to export a single class, function or primitive from a script file. The export can also be written as export default class HelloWorld extends React.Component { render() { return <p>Hello, world!</
Why export default is not working?
The "export default was not found" error occurs when we try to import as default from a module that doesn't have a default export. To solve the error, make sure the module has a named export and wrap the import in curly braces, e.g. import {myFunction} from './myModule' .
What is named export in React?
What are Named Exports? Named exports allow us to share multiple objects, functions or variables from a single file and were introduced with the release of ES2015. Named exports are imported with curly braces in various files and must be imported using the name of the object, function or variable that was exported.
Can I have two export default?
You can have only one default export per file, but you can have as many named exports as necessary. If you don't want to use a named export, move the second variable to a separate file and make sure to stick to a maximum of 1 default export per file.
How do I export a function in TypeScript?
Use named exports to export a function in TypeScript, e.g. export function sum() {} . The exported function can be imported by using a named import as import {sum} from './another-file' . You can use as many named exports as necessary in a single file.
How do I export default node JS?
exports = add; console. log(module); In the above code, Rectangle class and add function are exported as default exports.
How do you call a function from an external JavaScript file?
Calling a function using external JavaScript file
Once the JavaScript file is created, we need to create a simple HTML document. To include our JavaScript file in the HTML document, we have to use the script tag <script type = "text/javascript" src = "function.
How do you call a function from one file to another in node JS?
To include functions defined in another file in Node. js, we need to import the module. we will use the require keyword at the top of the file. The result of require is then stored in a variable which is used to invoke the functions using the dot notation.
How do you pass value from one js file to another in React?
For passing the data from the child component to the parent component, we have to create a callback function in the parent component and then pass the callback function to the child component as a prop. This callback function will retrieve the data from the child component.
References
- https://www.myboosting.gg/blog/apex-legends/apexlegends-romances-relationships
- https://www.dexerto.com/apex-legends/best-legends-to-use-in-apex-legends-ultimate-tier-list-1194717/
- https://www.washingtonpost.com/technology/2022/03/09/police-technologies-future-of-work-drones-ai-robots/
- https://www.geeksforgeeks.org/reactjs-defaultprops/
- https://www.droneblog.com/how-to-spot-a-drone-at-night-things-to-look-for/
- https://www.dexerto.com/apex-legends/apex-legends-writer-reveals-new-lore-details-for-octane-mirage-1346714/
- https://www.glassdoor.co.in/Compare/Apple-vs-Google-EI_IE1138-E9079.htm
- https://www.dictionary.com/browse/seer
- https://www.bbc.co.uk/news/technology-12343597
- https://www.forbes.com/sites/theyec/2019/01/29/looking-to-get-into-blockchain-here-are-four-ways-to-get-involved/
- https://www.thegamer.com/apex-legends-seer-resurgence/
- https://apexlegends.fandom.com/wiki/Pathfinder
- https://www.dexerto.com/apex-legends/apex-legends-pro-albralelie-explains-why-seer-is-gaming-breaking-in-current-meta-1875899/
- https://www.simplilearn.com/blockchain-programming-languages-article
- https://www.seeker.com/how-much-of-the-internet-is-hidden-1792697912.html
- https://www.expressvpn.com/blog/4-ways-to-hide-from-drone-surveillance/
- https://gamerant.com/apex-legends-seer-tips-guide/
- https://answers.ea.com/t5/Bug-Reports/Seer-s-tactical-and-Lifeline-s-revive/td-p/10572346
- https://www.dorset.police.uk/support-and-guidance/safety-in-your-community/use-of-drones/
- https://www.ambitionbox.com/profile/blockchain-developer-salary
- https://twitter.com/preslyy_/status/1552058876618428416
- https://bobbyhadz.com/blog/react-import-variable-from-another-file
- https://apexlegends.fandom.com/wiki/Gibraltar
- https://www.bbc.com/news/business-42060091
- https://www.zdnet.com/article/best-browser-for-privacy/
- https://www.radiusits.com/blog/microsoft-or-google/
- https://gloot.com/blog/outlands-news-2-secret-identities-family-drama-and-more
- https://www.indeed.com/companies/compare/Google-vs-Microsoft-d5cd619626c9f6f7-e3a48f8a4a788271
- https://lgbtqia-characters.fandom.com/wiki/Amity_Blight
- https://hr.lib.byu.edu/00000179-1484-d8b8-a1fd-5496279e0000/werewolf-game
- https://www.stanleyulili.com/node/node-modules-import-and-use-functions-from-another-file/
- https://www.asuraworld.com/articles/How%20to%20Counter%20Seer%20in%20Apex%20Legends
- https://stackoverflow.com/questions/21117160/what-is-export-default-in-javascript
- https://yourdronereviews.com/drones-for-law-enforcement
- https://www.businessinsider.in/stock-market/top-market-cap-companies-in-the-world/slidelist/93952579.cms
- https://fitsmallbusiness.com/g-suite-vs-office-365/
- https://www.careerera.com/blog/which-is-better-blockchain-or-data-science
- https://stackoverflow.com/questions/36426521/what-does-export-default-do-in-jsx
- https://tracker.gg/apex/leaderboards/stats/all/SeasonWins
- https://gamerant.com/apex-legends-season-13-character-tier-list-newcastle/
- https://www.dexerto.com/apex-legends/seer-heirloom-apex-legends-1973778/
- https://www.educative.io/answers/what-are-module-exports-in-javascript
- https://dronesgator.com/can-drones-see-inside-your-house-through-walls-or-curtains/
- https://www.insiderintelligence.com/content/google-remains-most-popular-us-search-engine
- https://www.rockpapershotgun.com/apex-legends-seer-abilities-tips-tricks
- https://www.trustedreviews.com/news/are-macs-safer-than-windows-laptops-4205593
- https://www.dexerto.com/apex-legends/how-to-play-seer-in-apex-legends-abilities-tips-more-1623325/
- https://www.indiatimes.com/technology/news/microsoft-beats-apple-to-become-worlds-most-valuable-company-again-552916.html
- https://www.cbr.com/apex-legends-wattson-gibraltar-season-7/
- https://www.geeksforgeeks.org/how-to-pass-data-from-one-component-to-other-component-in-reactjs/
- https://murder-mystery-2.fandom.com/wiki/Random_Painted_Seers
- https://www.upgrad.com/blog/top-10-highest-paying-jobs-in-india/
- https://argoblockchain.com/articles/bitcoins-undeniable-mathematics
- https://en.wikipedia.org/wiki/Google_services_outages
- https://www.inverse.com/gaming/apex-legends-season-10-seer-release-date-abilities-story-voice-actor-gameplay
- https://www.cnet.com/tech/services-and-software/what-would-it-take-to-beat-google/
- https://www.gamespot.com/articles/apex-legends-seer-can-see-heartbeats-farther-than-he-can-hear-them/1100-6494593/
- https://www.vocabulary.com/dictionary/seer
- https://www.einvestigator.com/the-use-of-drones-in-law-enforcement-and-private-investigation/
- https://www.gadgetreview.com/how-far-can-military-drones-fly
- https://en.wikipedia.org/wiki/Don%27t_be_evil
- https://hired.com/job-roles/blockchain-engineer
- https://california.universitypressscholarship.com/view/10.1525/california/9780520252295.001.0001/upso-9780520252295-chapter-8
- https://www.investopedia.com/news/public-private-permissioned-blockchains-compared/
- https://www.macrotrends.net/stocks/charts/SEER/seer/net-worth
- https://liquipedia.net/apexlegends/Portal:Statistics
- https://seekingalpha.com/article/4550059-google-vs-microsoft-which-is-the-better-choice
- https://www.thegamer.com/apex-legends-character-roster-age-height-home-world/
- https://www.ambitionbox.com/salaries/microsoft-corporation-salaries
- https://vikings.fandom.com/wiki/The_Seer
- https://www.dexerto.com/apex-legends/apex-legends-octane-seer-season-15-map-teaser-1964285/
- https://answers.ea.com/t5/General-Discussion/Seer-or-crypto-who-s-currently-the-better-legend/td-p/10911131
- https://mybabysittersavampire.fandom.com/wiki/Seer
- https://www.droneblog.com/drone-camera-distance/
- https://www.oldest.org/entertainment/youngest-apex-legend-characters/
- https://twitter.com/fanbytemedia/status/1100173821733203968
- https://www.heliguy.com/blogs/posts/five-ways-police-are-using-drones
- https://www.analyticsinsight.net/how-to-become-a-self-taught-blockchain-developer-and-earn-millions/
- https://www.cnet.com/tech/services-and-software/bill-gates-defends-bing-and-windows-8/
- https://blog.prepscholar.com/what-comes-after-trillion
- https://www.upgrad.com/blog/prerequisites-to-learn-blockchain/
- https://www.geeksforgeeks.org/different-job-roles-in-blockchain-technology/
- https://www.dexerto.com/apex-legends/wattson-players-call-for-buff-in-apex-legends-to-counter-seer-1625363/
- https://www.caa.co.uk/consumers/remotely-piloted-aircraft/general-guidance/reporting-misuse-of-a-unmanned-aircraft-and-drones/
- https://www.linkedin.com/pulse/why-blockchain-jobs-careers-future-jesse-anglen
- https://afkgaming.com/esports/news/tsm-imperialhal-hits-number-1-predator-on-apex-legends
- https://gloot.com/blog/apex-legends-lifeline-the-combat-medic
- https://tvtropes.org/pmwiki/pmwiki.php/Main/BlindSeer
- https://dotesports.com/apex-legends/news/everything-we-know-about-seer-in-apex-legends
- https://blog.imarticus.org/is-mathematics-required-to-implement-blockchain-solutions-in-business-fintech-blog/
- https://www.esportstales.com/apex-legends/most-played-characters-and-tier-list
- https://www.west-midlands.police.uk/frequently-asked-questions/police-drones
- https://dronereviewsplace.com/why-are-drones-following-me/
- https://seekingalpha.com/article/4523185-apple-vs-google-stock-clear-winner
- https://www.dexerto.com/apex-legends/imperialhal-explains-why-seer-is-broken-and-makes-apex-legends-easy-mode-1848499/
- https://www.911security.com/en-us/knowledge-hub/drone-detection/radar
- https://www.statista.com/statistics/234529/comparison-of-apple-and-google-revenues/
- https://www.polygon.com/22638438/apex-legends-update-patch-notes-seer-nerf-passive
- https://faculty.math.illinois.edu/~castelln/prillion_revised_10-05.pdf
- https://www.thestar.com/business/opinion/2022/02/19/google-search-has-gotten-worse-heres-the-trick-people-have-found-to-get-around-it.html
- https://www.ea.com/games/apex-legends/about/characters/wattson
- https://dronereviewsplace.com/how-far-can-drones-go/
- https://en.wikipedia.org/wiki/Apple_Computer,_Inc._v._Microsoft_Corp.
- https://www.sanfoundry.com/cplusplus-programming-questions-answers-functions/
- https://www.knowledgehut.com/blog/blockchain/programming-language-for-blockchain-development
- https://www.w3schools.com/react/react_es6_modules.asp
- https://coptrz.com/blog/drone-solutions-for-police-how-are-the-police-using-drones/
- https://panmore.com/google-swot-analysis-recommendations
- https://www.investopedia.com/articles/personal-finance/042415/story-behind-googles-success.asp
- https://www.delftstack.com/howto/react/export-default-in-react/
- https://www.911security.com/en-us/knowledg-hub/drone-detection
- https://www.dexerto.com/apex-legends/all-apex-legends-heirlooms-how-to-get-heirloom-shards-1510719/
- https://dotesports.com/apex-legends/news/best-legends-to-pair-with-seer-in-apex
- https://medium.com/blockworks-group/is-blockchain-better-than-a-database-d518743bdafa
- https://science.howstuffworks.com/drone-spying.htm
- https://www.gamespot.com/articles/seer-guide-apex-legends/1100-6494808/
- https://seekingalpha.com/article/4542480-google-vs-tesla-which-stock-better-forecast
- https://interestingengineering.com/culture/what-would-happen-if-google-suddenly-stopped-working
- https://www.esportstales.com/apex-legends/characters-official-ages
- https://www.eurogamer.net/hands-on-with-wattson-the-newest-apex-legend
- https://www.ggrecon.com/guides/apex-legends-seer-nerf/
- https://nocamels.com/2022/03/israel-see-through-wall-game-changer/
- https://www.inc.com/jt-odonnell/6-reasons-working-at-google-isnt-right-for-most-people.html
- https://www.blockchain-council.org/blockchain/java-or-python-which-suits-blockchain-better/
- https://bobbyhadz.com/blog/javascript-import-function-from-another-file
- https://www.businessinsider.com/25-giant-companies-that-earn-more-than-entire-countries-2018-7
- https://www.simplilearn.com/how-to-start-a-career-in-blockchain-technology-article
- https://blockchaintrainingalliance.com/blogs/news/the-5-highest-paying-blockchain-jobs-in-2022
- https://www.impression.co.uk/blog/bing-differ-google/
- https://www.upgrad.com/blog/skills-needed-to-become-blockchain-developer/
- https://www.nytimes.com/1992/06/28/business/microsoft-s-unlikely-millionaires.html
- https://titanfall.fandom.com/f/p/3300297438920853131
- https://bobbyhadz.com/blog/react-import-function-from-another-file
- https://en.wikipedia.org/wiki/Microsoft
- https://www.cnet.com/tech/services-and-software/googles-enemy-list-a-primer/
- https://blog.hubspot.com/marketing/top-search-engines
- https://afkgaming.com/esports/guide/seer-vs-bloodhound-who-is-the-better-recon-legend
- https://scufgaming.com/gaming/apex-legends/apex-legends-character-tier-list-ranked
- https://bobbyhadz.com/blog/typescript-a-module-cannot-have-multiple-default-exports
- https://screenrant.com/vikings-show-seer-hand-lick-characters-reason-explained/
- https://nordvpn.com/blog/private-search-engines/
- https://www.bundleapps.io/blog/use-named-exports-over-default-exports-in-javascript
- https://virgin-vs-chad.fandom.com/wiki/Wraith
- https://www.dexerto.com/apex-legends/seer-sees-apex-legends-pick-rate-collapse-after-major-nerfs-1641013/
- https://gamerant.com/apex-legends-character-story/
- https://www.computerworld.com/article/2492642/microsoft-s-office-365-home-premium-to-cost--99-99-annually-per-subscription.html
- https://www.dualshockers.com/roblox-murder-mystery-2-mm2/
- https://www.dronerush.com/best-long-range-drones-18975/
- https://www.gamespot.com/articles/apex-legends-season-15-launch-trailer-reveals-catalyst-and-seer-have-some-serious-beef/1100-6508472/
- https://www.droneblog.com/drone-following-me/
- https://www.comparably.com/blog/study-what-its-like-to-interview-amazon-apple-facebook-google-microsoft/
- https://apexlegends.fandom.com/wiki/Seer_(character)
- https://gloot.com/blog/apex-legends-how-to-really-pick-your-main
- https://california.universitypressscholarship.com/view/10.1525/california/9780520252295.001.0001/upso-9780520252295-chapter-2
- https://betterprogramming.pub/understanding-the-difference-between-named-and-default-exports-in-react-2d253ca9fc22
- https://cultofdrone.com/drone-laws-in-the-uk/
- https://www.blockchain-council.org/blockchain/how-can-a-newbie-start-learning-about-blockchain/
- https://www.techtarget.com/whatis/definition/googol-and-googolplex
- https://apexlegends.fandom.com/wiki/Valkyrie
- https://www.rockpapershotgun.com/apex-legends-next-character-seer-is-cursed-with-a-deadly-gaze
- https://bobbyhadz.com/blog/typescript-export-function
- https://apexlegends.fandom.com/wiki/Seer/Voice_lines
- https://phys.org/news/2010-02-internet-sites-google-people-smarter.html
- https://www.charlieintel.com/apex-legends-seer-lil-nas-x/119896/
- https://www.coursera.org/collections/learn-blockchain
- https://www.hackreactor.com/blog/top-companies-paying-software-engineers-the-most-in-2022
- https://www.javatpoint.com/how-to-call-javascript-function-in-html
- https://apexlegends.fandom.com/wiki/Fuse
- https://apexlegendsstatus.com/leaderboard/Seer/kills/1
- https://escapethewolf.com/2296/when-you-under-physical-surveillance/
- https://murder-mystery-2.fandom.com/wiki/Seer
- https://fifthperson.com/apple-vs-microsoft/
- https://www.glassdoor.com/Compare/Microsoft-vs-Google-EI_IE1651-E9079.htm
- https://www.udacity.com/course/blockchain-developer-nanodegree--nd1309
- https://www.cnet.com/tech/tech-industry/artificial-intelligence-is-no-smarter-than-a-six-year-old-study-says/
- https://www.dexerto.com/apex-legends/apex-legends-characters-age-history-1866017/
- https://www.tutorialsandyou.com/nodejs/what-is-export-default-in-nodejs-2.html
- https://computersciencehero.com/careers/blockchain-developer/
- https://www.careerera.com/blog/is-blockchain-a-good-career
- https://www.springboard.com/blog/software-engineering/highest-paying-programming-jobs/
- https://www.dexerto.com/apex-legends/apex-legends-devs-explain-no-seer-nerfs-despite-dominance-1938472/
- https://www.dexerto.com/apex-legends/which-characters-are-lgbtqia-in-apex-legends-1569544/
- https://www.gamespot.com/articles/apex-legends-stories-from-the-outlands-explains-why-lifeline-traded-family-for-a-punk-band/1100-6504588/
- https://www.wisestamp.com/blog/gmail-vs-outlook/
- https://dronesgator.com/can-drones-hear-conversations/
- https://gloot.com/blog/apex-legends-everything-you-need-to-know-about-bloodhound
- https://www.educative.io/answers/what-are-react-functional-components
- https://www.droneblog.com/drone-looks-from-ground/
- https://careerkarma.com/blog/how-to-get-a-job-in-blockchain/
- https://www.rockpapershotgun.com/best-apex-legends-characters-legend-tier-list-abilities-tips
- https://dronereviewsplace.com/why-are-there-drones-in-the-sky-at-night/
- https://www.geeksforgeeks.org/node-js-export-module/
- https://www.upgrad.com/blog/is-blockchain-a-bright-career-opportunity-for-non-techies-too/
- https://www.cbr.com/apex-legends-lore-ships/
- https://www.codingem.com/export-multiple-functions-in-javascript/
- https://www.zdnet.com/article/office-365-vs-g-suite-which-productivity-suite-is-best-for-your-business/
- https://stackoverflow.com/questions/66737429/export-function-inside-functional-component-in-react
- https://www.simplilearn.com/tutorials/blockchain-tutorial/how-to-become-a-blockchain-developer
- https://support.google.com/assistant/thread/134740704/how-can-i-turn-on-google-asstistants-offensive-words?hl=en
- https://www.mcafee.com/en-in/safe-browser.html
- https://www.visualcapitalist.com/ranked-the-most-valuable-brands-in-the-world/
- https://www.statista.com/statistics/216573/worldwide-market-share-of-search-engines/
- https://www.eccouncil.org/programs/blockchain-certification-courses/
- https://www.comparitech.com/privacy-security-tools/blockedinchina/bing/
- https://stackoverflow.com/questions/36866718/when-to-use-export-default-and-export-constant-in-javascript
- https://www.dexerto.com/apex-legends/apex-legends-dev-confirms-time-is-coming-for-major-seer-nerfs-2000288/
- https://apexlegends.fandom.com/wiki/Revenant
- https://www.eurogamer.net/apex-legends-seer-abilities-explained-launch-skins-list-8022
- https://www.oberlo.com/blog/top-search-engines-world
- https://screenrant.com/why-the-seer-really-licked-flokis-hand-in-vikings-season-4/
- https://bettermarketing.pub/how-tiktok-overtook-google-as-the-worlds-most-popular-website-d0fc79853c0a
- https://en.wikipedia.org/wiki/List_of_largest_companies_by_revenue
- https://www.droneblog.com/i-just-found-a-drone-what-should-i-do-with-it/
- https://bobbyhadz.com/blog/javascript-export-default-was-not-found
- https://nordvpn.com/blog/ios-vs-android-security/
- https://blog.prepscholar.com/googol-googolplex
- https://bobbyhadz.com/blog/react-export-multiple-functions
- https://www.xdynamics.com/blog/how-far-can-a-drone-fly/
- https://ntelt.cikd.ca/top-5-search-engines-used-in-daily-life/
- https://www.ggrecon.com/guides/apex-legends-seer-abilities-lore/
- https://murder-mystery-2.fandom.com/wiki/Purple_Seer
- https://www.eff.org/deeplinks/2022/01/how-are-police-using-drones
- https://www.ign.com/wikis/apex-legends/Seer_Guide_and_Tips
- https://gamerant.com/apex-legends-characters-ranked-difficulty/
- https://apexlegends.fandom.com/wiki/Seer
- https://dronesgator.com/how-to-see-a-drone-at-night/